We Cover #7 and #8 today
For #1 to # 6, please visit: Basics to Practice of PHP in A Week -1
- What is PHP
- Environment Setup
- Basic Structure of the Program
- Execution Method
- Variables
- Data types
- Arrays
- Operators
- Branch Processing
- Iteration
- Function
- Method
- Class
- Practice
Arrays
An array is a data type that can store multiple data.
An array with one column is called a one-dimensional array, and an array with two or more columns is called a multidimensional array.
Each box in the array is called an element, and the number of boxes is called the number of elements.
Location information is assigned to each element of the array.
It’s like the address where the data lives.
This address is assigned a number called an index.
The index is also called “subscript”.
The leftmost index starts at 0. Note that it does not start from 1.
Therefore, the first index is “0”, the second index is “1”, the third is “2”, the fourth is “3”, and so on. “
How to make an array -1
There are several ways to create an array, but I will introduce two of them.
Variable = array (number of elements);
First, define the number of arrays, and then put a value in each index.
<?php
$friends = array(3);
$friends[0] = "Kojima";
$friends[1] = "Koike";
$friends[2] = "Kawai";
echo ($friends[0])."\n";
echo ($friends[1])."\n";
echo ($friends[2])."\n";
Returns in index order as shown below.

You can also write elements in parentheses without specifying the number of variables.
<?php
$friends = array("Kojima", "Koike", "Kawai");
echo ($friends[0])."\n";
echo ($friends[1])."\n";
echo ($friends[2])."\n";
How to make an array -2
The second is the method using array literals.
Array variable = [Data 1, Data 2,….];
This way of writing is called array literal initialization.
<?php
$family = ["mother", "father", "sister"];
echo ($family[0])."\n";
echo ($family[1])."\n";
echo ($family[2])."\n";

How to change the elements of an array
<?php
$family = ["mother", "father", "sister"];
$family[2] = "brother"; ## Add this code
echo ($family[0])."\n";
echo ($family[1])."\n";
echo ($family[2])."\n";
You can change the element by specifying an index.

Multidimensional array
In the case of a two-dimensional array, write the parentheses inside the parentheses and describe the data to be assigned there.
<?php
$food = [["apple", "banana"],["tomato", "cabbage"]];
echo ($food[0][0])."\n"; ## It is [0][0] because it is the first in the first array.
echo ($food[0][1])."\n"; ## It is [0][1] because it is the second of the first array.
echo ($food[1][0])."\n"; ## It is [1][0] because it is the first in the second array.
echo ($food[1][1])."\n"; ## It is [1][1] because it is the second of the second array.
Returns in the specified order as shown below.
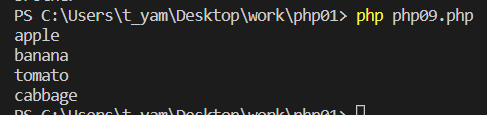
Operators
An operator is a symbol used for four arithmetic operations such as addition and subtraction, and when comparing two values.
Arithmetic operator
<?php
$x = 100;
$y = 3;
echo ($x + $y)."\n";
echo ($x - $y)."\n";
echo ($x * $y)."\n";
echo ($x / $y)."\n";
echo ($x % $y)."\n";
% returns the remainder when x is divided by y.
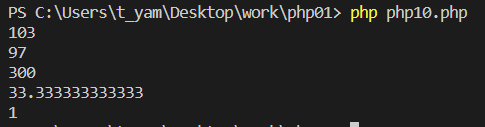
Relational operator
A relational operator is an operator that makes the relationship between two values determine true or false.
“1” is returned when the relationship between the two is correct.
<?php
$x = 100;
$y = 3;
$z = 100;
echo ($x > $y)."\n";
echo ($x >= $z)."\n";
echo ($x < $y)."\n";
echo ($x <= $z)."\n";
Below, only echo ($ x <$ y). “\ N”; is false, so nothing is returned, but otherwise it is true, so 1 is returned.
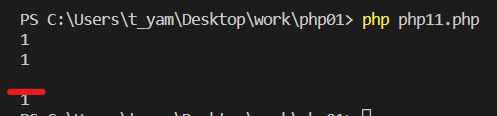
Equivalence is two equals.
If they are not equivalent, they are equal to the exclamation mark.
<?php
$x = 100;
$y = 3;
$z = 100;
echo ($x > $y)."\n"; ## Returns "1" if x is greater than y
echo ($x == $z)."\n"; ## Returns "1" if x and z are equal
echo ($x != $z)."\n"; ## Returns "1" if X and z are NOT equal
echo ($x < $y)."\n"; ## Returns "1" if x is less than y
echo ($y != $z)."\n"; ## Returns "1" if y and z are NOT equal
Below, the expected results are returned.
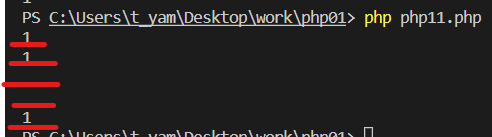
Logical operator
A logical operator is an operator that determines multiple conditions.
The “and condition” has two &&, and the “or condition” has two vertical lines || (pipeline).
For example, the number “100” is greater than or equal to 5 and less than or equal to 200.
The number “30” is more than 5 and not less than 10.
<?php
$x = 100;
$y = 30;
echo ($x >= 5 && $x <= 200)."\n";
echo ($y >= 5 && $y <= 10)."\n";
The first is True and the second is False, so the response is as expected.

When the previous “and condition” is changed to “or condition”, if either one is True, “1” is returned.
<?php
$x = 100;
$y = 30;
echo ($x >= 5 || $x <= 200)."\n";
echo ($y >= 5 || $y <= 10)."\n";
Below, both are returning “1”.

Assignment operator
The “=” used when assigning to a variable is called an assignment operator.
Also, when assigning, the operator that assigns in combination with addition, subtraction, etc. is called the compound assignment operator.
For example, the method of adding “5” to the variable x and then assigning it to x is “x + = 5”.
For example, the method of adding y to z and then assigning it to z is “z + = y”.
<?php
$x = 10;
$y = 20;
$z = 300;
echo ($x += 4)."\n";
echo ($z += $y)."\n";
The first formula is 10 (x) plus 4, the answer is 14, the second is 20 (y) plus 300 (z), and the answer is 320.

Increment and decrement
Increment is an operator that increases the value by “1” and is written as “variable name ++“.
For example, the way to add “1” to x is “x ++“.
This has the same meaning as “x = x + 1“.
Decrement is an operator that decrements the value by “1” and writes “variable name–“.
For example, the way to write y to reduce “1” is “y–“.
This has the same meaning as “y = y-1“.
<?php
$x = 100;
$y = 100;
$x++;
$y--;
echo ($x)."\n";
echo ($y)."\n";
Since 1 is added to “x” and 1 is subtracted from “y”, 101 and 99 are returned, respectively.
