We’ll cover #9 to #10
#1 to #6 is HERE: Basics to Practice of PHP in A Week -1
#7 & #8 is HERE: Basics to Practice of PHP in A Week -2
- What is PHP
- Environment Setup
- Basic Structure of the Program
- Execution Method
- Variables
- Data types
- Arrays
- Operators
- Branch Processing
- Iteration
- Function
- Method
- Class
- Practice
Conditional branch
A conditional branch can be called “process A” if the condition is met, and “process B” if it is not.
if-else statement
Let age be 18. The condition of the if statement is 20 or older. If the age is 20, “You can drink.”
Otherwise, it is a conditional branch that displays “You cannot drink.”
<?php
$age = 18;
if ($age > 20) {
echo("You can drink");
} else {
echo("You cannot drink");
}
This time the age is 18, so “You cannot drink.” Is returned.

Let’s change the age to 33 as follows.
<?php
$age = 33;
if ($age > 20) {
echo("You can drink");
} else {
echo("You cannot drink");
}
The message has changed to “You can drink”.

if ~ else if ~ else statement
Returns “Don’t drink too much” if you are the age is 20 or older and 50 or younger, “Stop drinking” if the age is 51 or older, and “You cannot drink” otherwise (19 or younger).
<?php
$age = 55;
if ($age >=20 && $age <=50) {
echo("Don't drink too much");
} else if ($age >=51){
echo("Stop drinking");
} else {
echo("You cannot drink");
}
The age is 55, sit returns “Stop drinking”.

Iteration
Repetition is a program structure that executes the same process if a fixed number of times and conditions are met.
for statement
The for statement repeats the same process if the conditions are met.
Then, when the conditions are no longer met, the repetition ends.
If you want to repeat the same process 5 times with a for statement, the code will be as follows.
<?php
for ($i = 0; $i <=4; $i ++){
echo $i."\n";
}
✅ for —– Beginning of Iteration
✅ i —– starting value (You can start any number you want)
✅ i <= 4 —– The for statement always specifies when it will end. If you forget this, you will end up in an infinite loop, so be careful.
✅ i ++ —– Increment operator. Adding 1 each time
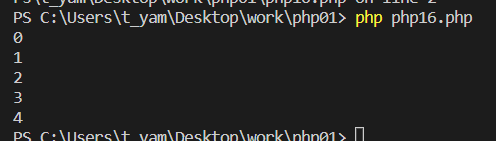
Start from zero, add 1 each, repeat 5 times, and finish with 4.
break
The break statement can end the iterative process when certain conditions are met.
For example, start from “0”, increase by 1, and end the repetition when it reaches “3”.
<?php
for($i = 0; $i <=4; $i ++) {
if($i == 3) {
break;
}
echo $i."\n";
}
The “start value i” is started from zero, and when i becomes 3, it is “break” (end of iterative processing).
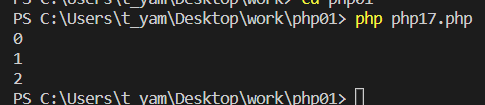
continue
The continue statement is used when you want to skip the process when a certain condition is met in the iterative process.
For example, if you start from “0” and increase by 1, then skip “3” when it reaches “3”.
<?php
for($i = 0; $i <= 4; $i ++) {
if($i == 3) {
continue;
}
echo $i."\n";
}
“Start value i” starts from zero, skips when “i” reaches 3, and ends at 4.
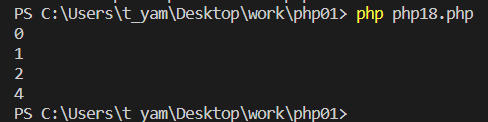
Nested “for statements”
You can also put a for statement inside a for statement.
Below example, the outer repeat counter variable is “i”, turning from 0 to 2, and the inner repeat counter variable is “j”, repeating from 0 to 2.
<?php
for($i = 0; $i <= 4; $i ++) {
for($j = 0; $j <= 4; $j ++){
echo $i. ': '.$j."\n";
}
}
When the “second for statement” is repeated 5 times in the “first for statement”, the second round “first for statement” starts.
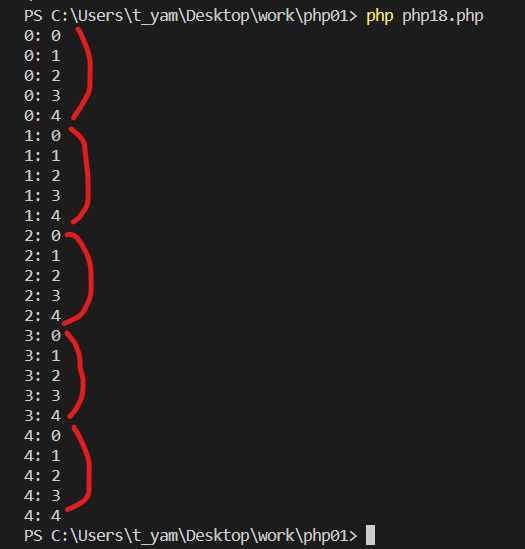
Refer to the array with a for statement
<?php
$myNum = [1,3,5,7,9];
$sum = 0;
for($i = 0; $i <= 4; $i++) {
echo $myNum[$i]."\n";
$sum += $myNum[$i];
}
echo $sum."\n";
The values of the array are stored in the variable “myNum” one by one from the beginning and displayed.
In addition, the variable “sum” is used to display the total of “25” in the array.
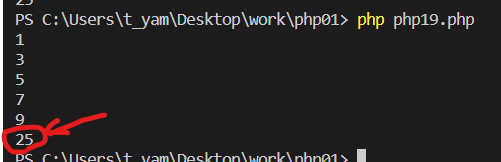