Folder structure
When routing Django’s website, always needs to be aware of the location of the templates you have placed in your project or app. This post introduces the basics of where to write routing and how to write it.
Project name: ‘report’
2 apps: ‘lesson’, ‘student‘
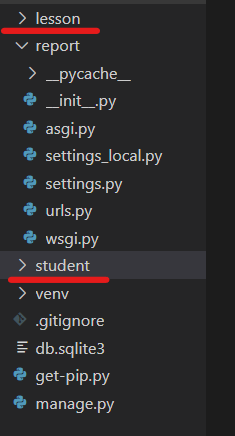
Student app controls user data and teachers will use lesson app for reporting each lesson
Lesson system:
- one on one lessons
- many (teacher) to many (student)
- courses divided by English-qualification (eiken) level
- Each course has several materials
- Each material has unit number and marked by teacher when completed in lesson
- The material is divided into four categories: reading, writing, listening, and speaking
- Students book lessons with different teachers on random dates and times
Overview of settings
Add 2 apps to INSTALLED_APPS on project.settings.py
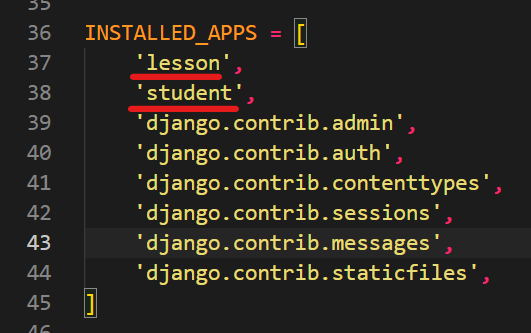
*To make the routing easier to understand, only the elements are shown without adding any design.
Templates Folders and HTML Files
App: student
student/templates/base.html
student/templates/registration/login.html
student/templates/student/index.html
student/templates/student/profile.html
student/templates/studet/student_list.html
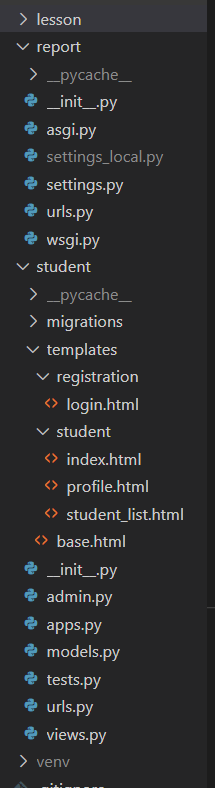
App: lesson
lesson/templates/lesson/report.html
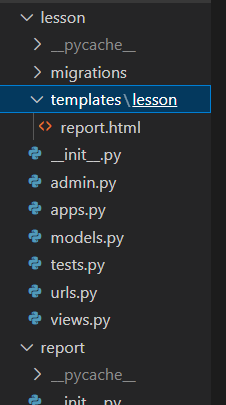
Associate app.urls.py with project.urls.py
Create urls.py for each app folder, and associate urls.py in the project folder with urls.py in the application folder.
The basic principle is to manage app addresses within the target app folder.
First, add below code to project.urls.py
By using “include”, you can associate project.urls.py with app.urls.py
from django.contrib import admin
from django.urls import path, include <--Add
urlpatterns = [
path('admin/', admin.site.urls), <-- This is for admin panel
path('', include('student.urls')), <-- Add
path('lesson/', include('lesson.urls')), <-- Add
]
Second, add below code to app1.urls.py
Create urls.py in the app1(student, in this case) folder and add code below.
Need to add “include” for adding “django.contrib.auth.urls” (login feature).
path(”, views.index, name=’index’)
= path(address to access, function to call, name=path name)
[from student import views] is to call views we’ll create later.
from django.urls import path, include
from student import views
urlpatterns = [
# Add django.contrib.auth
path("accounts/", include("django.contrib.auth.urls")),
# Add path to index
path('', views.index, name='index'), <-- 1st page visitors will see
path("profile/", views.profile, name="profile"), <-- Student Profile Page
path("student_list/", views.student_list, name="student_list"), <-- Student List for Teachers
]
Next, add below code to app2.urls.py
Again, create urls.py in the app2(lesson, in this case) folder and add code below.
[from lesson import views] is to call views we’ll create later.
from django.urls import path
from lesson import views
app_name = 'lesson'
urlpatterns = [
path("lesson/report", views.report, name="report"),
]
Add views (functions) to be called to views.py
Added a context dictionary and set the value to [index].
The render function is written with the request.
Since the template directory is automatically recognized by django, it is specified as student/index.html directly below.
Be careful not to include the templates directory in your render function.
from django.shortcuts import render
def index(request):
context = {
'message' : 'Saturday classes added',
'news' : '24 students passed Eiken grade-1',
}
return render(request, 'student/index.html', context)
def profile(request):
return render(request, "student/profile.html")
def student_list(request):
return render(request, "student/student_list.html")
The index.html contains the following code. (design will be added later)
{% extends ‘base.html’ %} needs to be on the first line for general settings.
Contents will be populated bestween {% block content %} and {% endblock %}.
User without login will see default setting –> Hello, {{ user.username | default:’Teacher’ }}!
To show different content by login status, set {% if user.is_authenticated %}{% else %}{% endif %}
Click here for login auth settings
<!-- Make sure add this code to send content to base.html -->
{% extends 'base.html' %}
<!-- Content on this template will be shown on base.html -->
{% block content %}
<!-- User without login will see this message -->
Hello, {{ user.username|default:'Teacher' }}!
<!-- Display different messages according to login status -->
<div>
{% if user.is_authenticated %}
<a href="{% url 'logout'%}">Logout</a>
{% else %}
<a href="{% url 'login' %}">Login</a>
{% endif %}
</div>
<!-- Populating message and news from index view -->
<ul>
<li>{{ message }}</li>
<li>{{ news }}</li>
</ul>
<!-- Redirecting to Student Profile and Student List pages -->
<div>
<p><a href="{% url 'profile' %}">Go to My Profile (profile.html)</a></p>
</div>
<div>
<p><a href="{% url 'student_list' %}">Go to Teacher Page (student_list.html)</a></p>
</div>
{% endblock %}
On the front end it looks like this depending on login status:
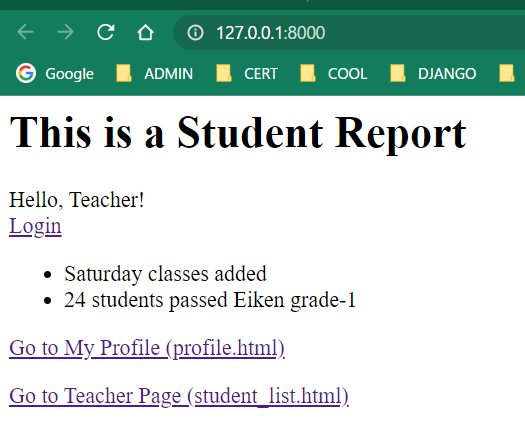
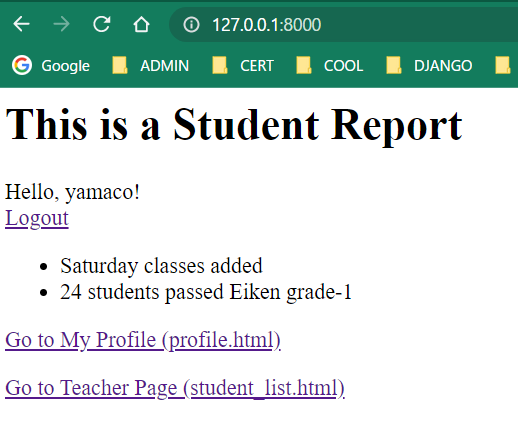
Click each link and see if routing is working
This time, the purpose is to set the routing, so the view settings and html are kept to a minimum.
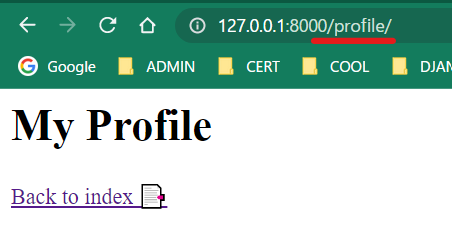
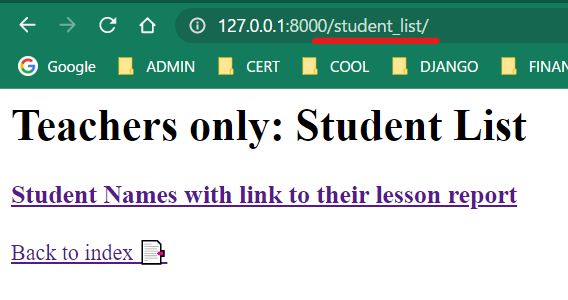
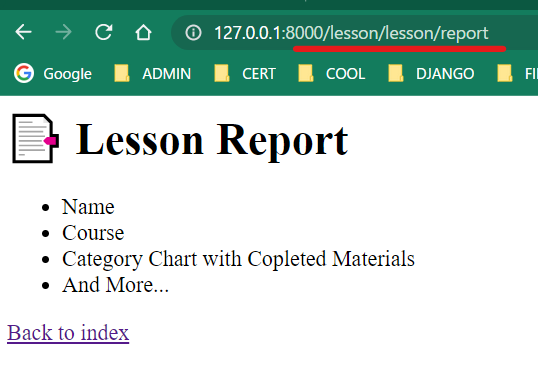