- Load the initial data of the student list with Django fixtures, Powershell, Notepad++
- Summary of This Topic
- Recommend
Load the initial data of the student list with Django fixtures, Powershell, Notepad++
With Powershell, data conversion can be done with two lines of code.
Create models.py
from django.db import models
class Students(models.Model):
id = models.AutoField(primary_key=True)
student_name = models.CharField(max_length=50, null=True, blank=True)
gender = models.IntegerField(choices=GENDER_CHOICES)
course_name = models.ForeignKey(Courses, on_delete=models.PROTECT, default=1)
created_at = models.DateTimeField(auto_now_add=True)
updated_at = models.DateTimeField(auto_now=True)
dob = models.DateField(max_length=8, null=True, blank=True)
def __str__(self):
return f"{self.id} | {self.student_name} | {self.gender} | {self.course_name}| {self.created_at} | {self.updated_at} | {self.dob} "
Create a “fixtures” folder in the app folder
Place the data you want to read in the folder created above.
Create “students.json” in “fixtures”
●The name of the json file is optional.
●Follow fixtures format ↓↓↓
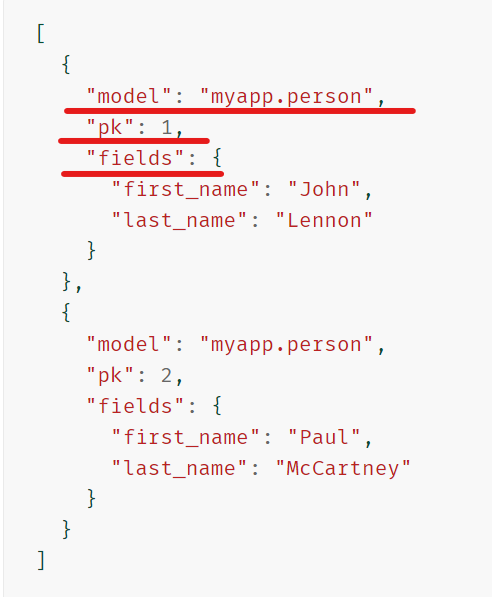
●Enter dummy data
[
{
"model": "students.students",
"pk": 1,
"fields": {
"student_name": "Miyabi Nakata",
"gender": "2",
"course_name": "1",
"created_at": "2020-7-20",
"updated_at": "2021-7-1",
"dob": "2000-3-3"
}
},
{
"model": "students.students",
"pk": 2,
"fields": {
"student_name": "Yushi Uno",
"gender": "1",
"course_name": "2",
"created_at": "2020-8-20",
"updated_at": "2021-8-1",
"dob": "2000-8-3"
}
}
]
Execute: python3 manage.py loaddata students.json
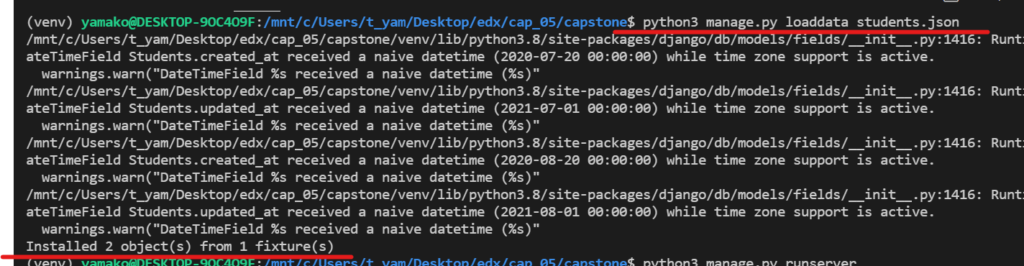
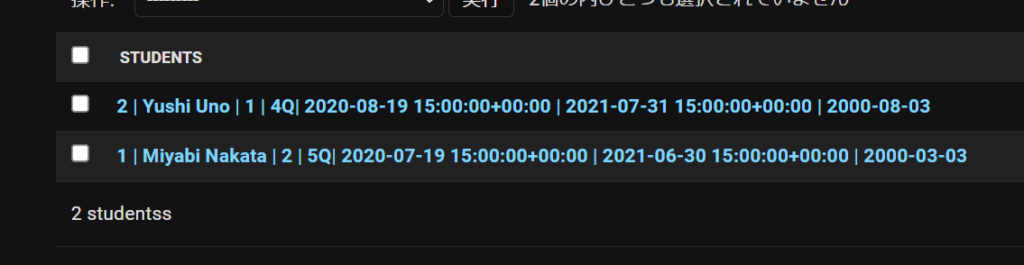
For large data loads:
Create a csv file of the table (data) to be read (name the columns the same as the keys in models.py)
(Try 4 cases for now.) All data are dummies.

Add a model to column A of the csv file
※ダミーデータ

Convert csv to json for import into database
Reference: Create Django Fixtures from Excel/CSV File
●When you open Powershell, first go to the csv folder and then just type the following two lines
PS C:\Users\t_yam\Downloads\LJ> $var = import-csv .\Students.csv | ConvertTo-Json
PS C:\Users\t_yam\Downloads\LJ> $var | Add-Content -Path "students.json"
●Now that the json file is created, open it in “Notepad++”.
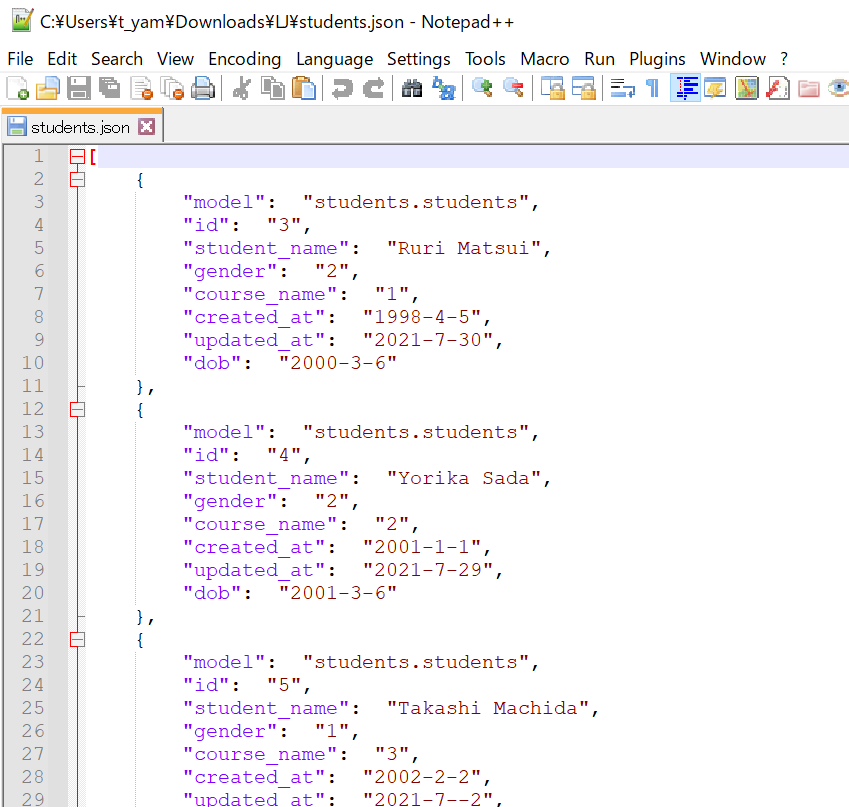
●Use replace to match the format of fixtures
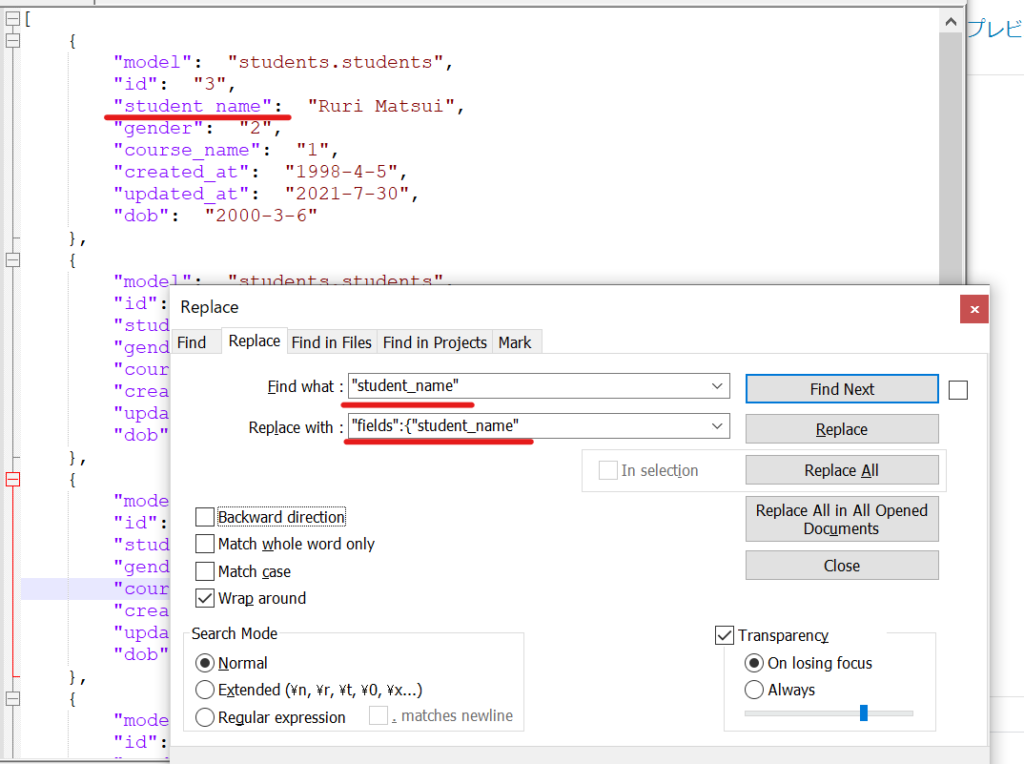
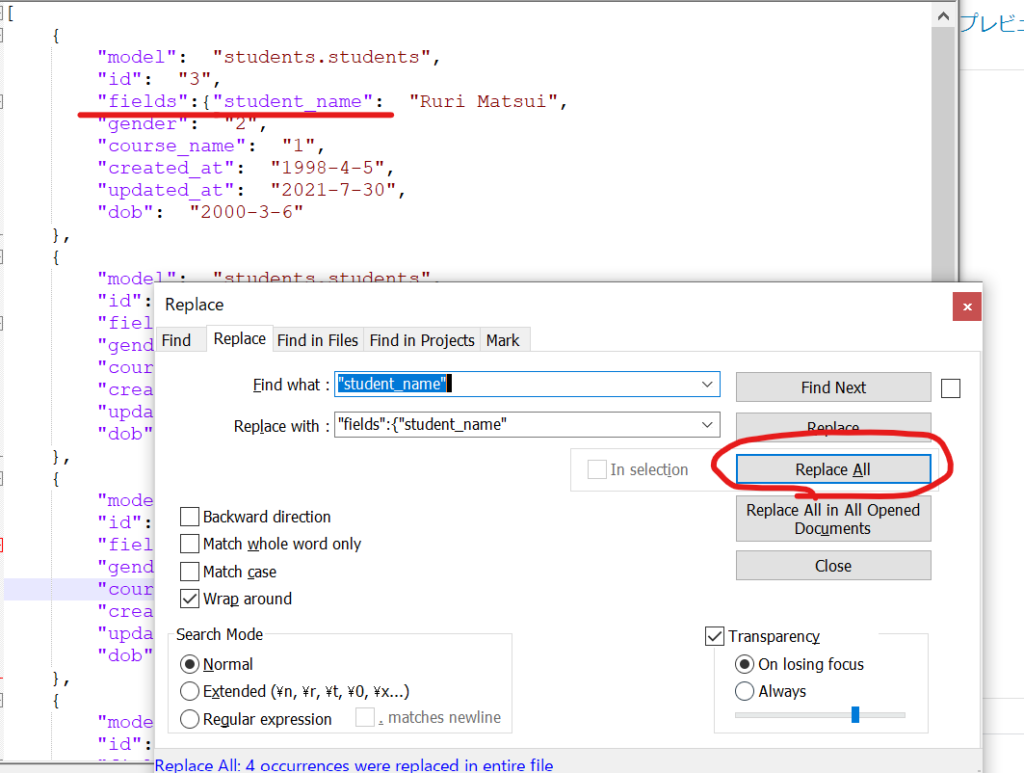
●Even closing brackets are converted at once.
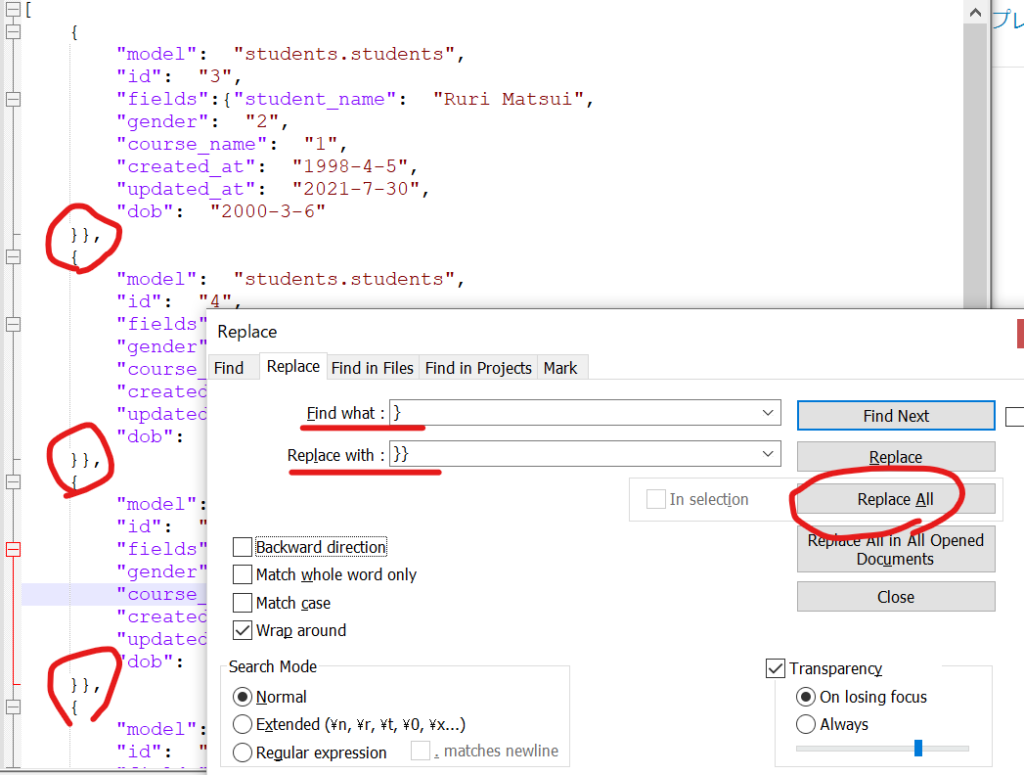
View the current database contents in the VSC extension sqlite
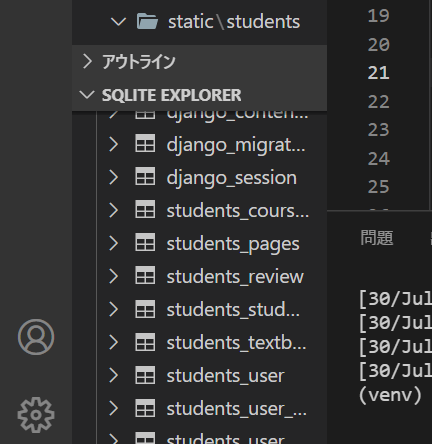
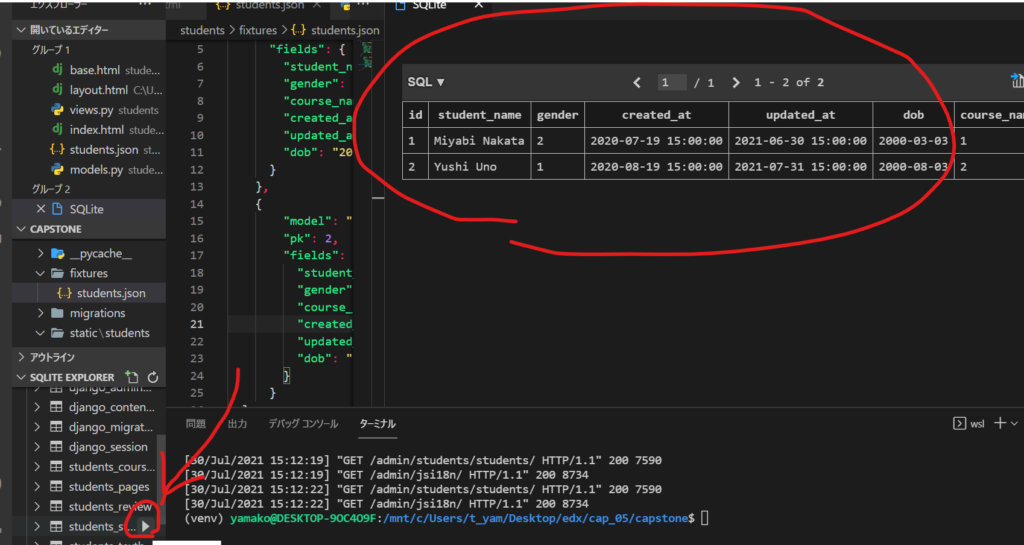
Currently there are 2 data registered (the dummy I just entered).
In production, I will replace the entire json file, but this time, I will add four new cases.
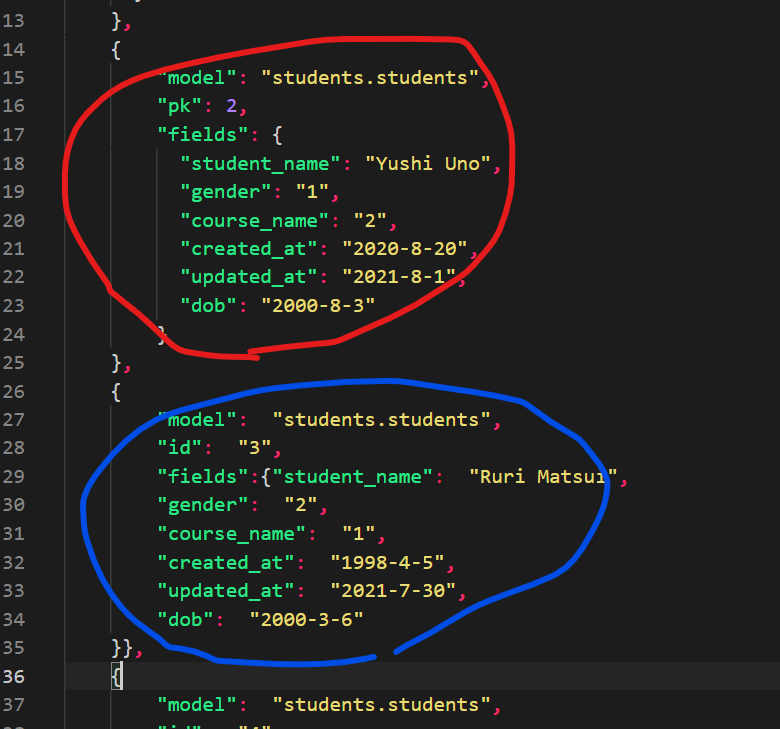
Add json data (blue) created with Notepad++ to the existing students.json (red) by copy and paste
Run again: python3 manage.py loaddata students.json
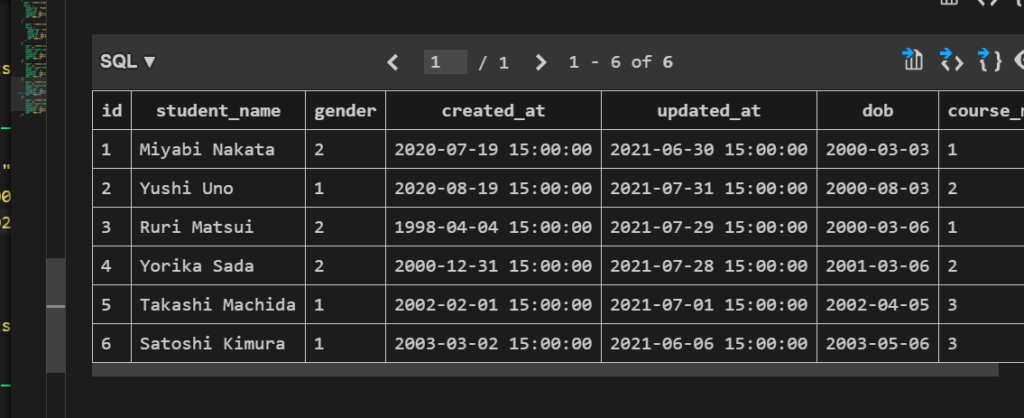
Successfully added four more!
Load the data from the previous section as a list (html).
{% extends "students/base.html" %}
{% block title %} Students {% endblock %}
{% block body %}
<div class="container">
<h2 style="text-align: center;">Student List</h2>
<table border="1">
<tr style="text-align: center;">
<th>id</th>
<th>name</th>
<th>1:M 2:F</th>
<th>course</th>
<th>Registered</th>
<th>Updated</th>
<th>BD</th>
</tr>
{% for student in object_list %}
<tr>
<td>{{ student.id }}</td>
<td>{{ student.student_name }}</td>
<td>{{ student.gender }}</td>
<td>{{ student.course_name }}</td>
<td>{{ student.created_at }}</td>
<td>{{ student.updated_at }}</td>
<td>{{ student.dob }}</td>
<th><a href="#">detail</a></th>
</tr>
{% endfor %}
</table>
</div>
{% endblock %}
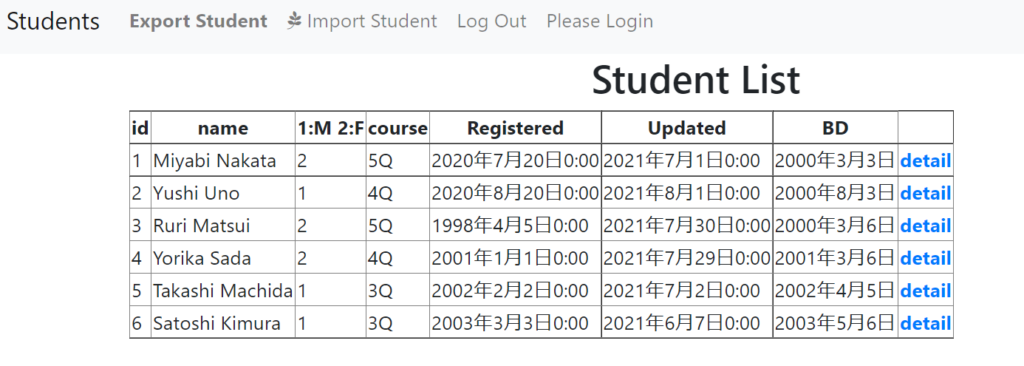
Initial data submission in Django
Creating initial data in Django with fixture
Summary of This Topic
When I typed the course name into the csv, it said, “It has to be a number!”, so once I entered a number (course ID). I will look into how to display the course name.